Hyperwave Magic Indicator
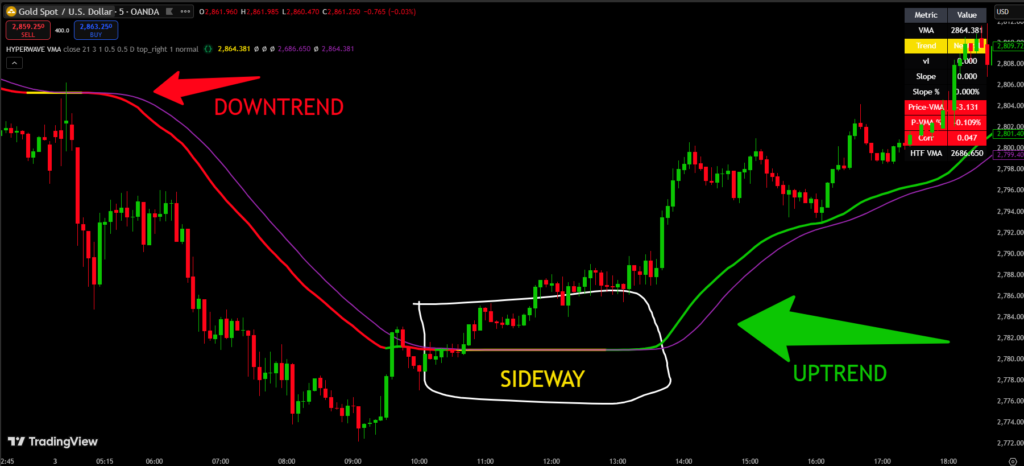
//@version=6
indicator('HYPERWAVE VMA]', shorttitle = 'HYPERWAVE VMA', overlay = true)
src = input(close, 'Source')
vmaLength = input.int(33, 'VMA Length', minval = 1)
showTrend = input.bool(true, 'Show Trend Colors')
barColorOpt = input.bool(false, 'Color Bars based on Trend')
lineWidth = input.int(3, 'Line Width', minval = 1)
upColor = input.color(color.new(color.orange, 0), 'Uptrend Color')
downColor = input.color(color.new(color.red, 0), 'Downtrend Color')
neutralColor = input.color(color.new(color.gray, 0), 'Neutral Trend Color')
fillArea = input.bool(true, 'Fill Area Between VMA and Price')
fillColor = input.color(color.new(color.gray, 80), 'Fill Color')
showPriceLine = input.bool(false, 'Plot Source Price as a Line', group = 'Additional Visuals')
priceLineColor = input.color(color.new(color.white, 0), 'Price Line Color', group = 'Additional Visuals')
priceLineWidth = input.int(1, 'Price Line Width', minval = 1, group = 'Additional Visuals')
showVolChannel = input.bool(true, 'Show Volatility Channel')
showSlopeLine = input.bool(true, 'Show VMA Slope Line')
showPivotPoints = input.bool(true, 'Show VMA Pivot Points')
showSuperVMA = input.bool(true, 'Show Super VMA (SMA of VMA)')
extremeSlopeThreshold = input.float(0.5, 'Extreme Slope (%)', step = 0.1)
lowCorrThreshold = input.float(0.5, 'Low Correlation Threshold', step = 0.1)
htfInput = input.timeframe('D', 'Higher Timeframe')
tablePosStr = input.string('top_right', 'Table Position', options = ['top_left', 'top_right', 'bottom_left', 'bottom_right'], group = 'Table Customization')
tableBorderWidth = input.int(1, 'Table Border Width', minval = 0, group = 'Table Customization')
tableHeaderBgColor = input.color(color.black, 'Table Header Background', group = 'Table Customization')
tableCellBgColor = input.color(color.new(color.black, 90), 'Default Table Cell Background', group = 'Table Customization')
tableTextColor = input.color(color.white, 'Table Text Color', group = 'Table Customization')
tableFontSizeStr = input.string('normal', 'Table Font Size', options = ['tiny', 'small', 'normal', 'large'], group = 'Table Customization')
tablePosition = tablePosStr == 'top_left' ? position.top_left : tablePosStr == 'bottom_left' ? position.bottom_left : tablePosStr == 'bottom_right' ? position.bottom_right : position.top_right
tableFont = tableFontSizeStr == 'tiny' ? size.tiny : tableFontSizeStr == 'small' ? size.small : tableFontSizeStr == 'large' ? size.large : size.normal
// VMA Calculation
k = 1.0 / vmaLength
pdm = math.max(src - src[1], 0)
mdm = math.max(src[1] - src, 0)
var float pdmS = pdm
pdmS := (1 - k) * nz(pdmS[1], pdm) + k * pdm
var float mdmS = mdm
mdmS := (1 - k) * nz(mdmS[1], mdm) + k * mdm
s = pdmS + mdmS
pdi = s != 0 ? pdmS / s : 0
mdi = s != 0 ? mdmS / s : 0
var float pdiS = pdi
pdiS := (1 - k) * nz(pdiS[1], pdi) + k * pdi
var float mdiS = mdi
mdiS := (1 - k) * nz(mdiS[1], mdi) + k * mdi
d = math.abs(pdiS - mdiS)
s1 = pdiS + mdiS
var float iS = 0.0
iS := s1 != 0 ? (1 - k) * nz(iS[1], d / s1) + k * (d / s1) : nz(iS[1], 0)
hhv = ta.highest(iS, vmaLength)
llv = ta.lowest(iS, vmaLength)
d1 = hhv - llv
vI = d1 != 0 ? (iS - llv) / d1 : 0
var float vma = src
vma := na(vma[1]) ? src : (1 - k * vI) * nz(vma[1]) + k * vI * src
// Additional Metrics
vmaSlope = vma - nz(vma[1])
slopePct = vma != 0 ? vmaSlope / nz(vma[1]) * 100 : 0
priceDiff = src - vma
priceDiffPct = vma != 0 ? priceDiff / vma * 100 : 0
superVMA = ta.sma(vma, 10)
vol = ta.stdev(src, vmaLength)
upperChannel = vma + vol
lowerChannel = vma - vol
htfVma = request.security(syminfo.tickerid, htfInput, vma)
corr = ta.correlation(src, vma, vmaLength)
// Primary Plotting
trendColor = vma > nz(vma[1]) ? upColor : vma < nz(vma[1]) ? downColor : neutralColor
pV = plot(vma, 'VMA', color = showTrend ? trendColor : neutralColor, linewidth = math.max(1, lineWidth))
pP = plot(showPriceLine ? src : na, 'Price', color = priceLineColor, linewidth = math.max(1, priceLineWidth))
fMain = fillArea and showPriceLine ? fillColor : na
fill(pV, pP, color = fMain)
barcolor(barColorOpt ? trendColor : na)
pU = plot(showVolChannel ? upperChannel : na, 'Upper Channel', color = color.new(upColor, 50), linewidth = 1)
pL = plot(showVolChannel ? lowerChannel : na, 'Lower Channel', color = color.new(downColor, 50), linewidth = 1)
fVol = showVolChannel ? color.new(neutralColor, 90) : na
fill(pU, pL, color = fVol)
plot(htfVma, 'HTF VMA', color = color.new(color.purple, 0), linewidth = 2, style = plot.style_circles)
bgcolor(math.abs(slopePct) >= extremeSlopeThreshold ? color.new(trendColor, 80) : na)
pS = plot(showSlopeLine ? ta.sma(vmaSlope, 5) : na, 'VMA Slope Line', color = color.new(color.blue, 0), linewidth = 2, style = plot.style_line)
ph = ta.pivothigh(vma, 3, 3)
pl = ta.pivotlow(vma, 3, 3)
plotshape(showPivotPoints and not na(ph) ? ph : na, 'Pivot High', style = shape.triangledown, location = location.abovebar, color = downColor, size = size.small)
plotshape(showPivotPoints and not na(pl) ? pl : na, 'Pivot Low', style = shape.triangleup, location = location.belowbar, color = upColor, size = size.small)
plot(showSuperVMA ? superVMA : na, 'Super VMA', color = color.new(color.purple, 0), linewidth = 2, style = plot.style_line)
// Dynamic Info Table
vmaCellColor = tableCellBgColor
trendCellColor = trendColor
viCellColor = tableCellBgColor
slopeCellColor = vmaSlope > extremeSlopeThreshold ? color.new(upColor, 0) : vmaSlope < -extremeSlopeThreshold ? color.new(downColor, 0) : tableCellBgColor
slopePctCellColor = math.abs(slopePct) >= extremeSlopeThreshold ? color.new(trendColor, 0) : tableCellBgColor
priceDiffCellColor = priceDiff > 0 ? color.new(upColor, 0) : priceDiff < 0 ? color.new(downColor, 0) : tableCellBgColor
priceDiffPctCellColor = priceDiffCellColor
corrCellColor = corr < lowCorrThreshold ? color.new(downColor, 0) : tableCellBgColor
htfVmaCellColor = tableCellBgColor
vmaTooltip = 'Variable Moving Average'
trendTooltip = 'Current Trend: Bullish/Bearish/Neutral'
viTooltip = 'Volatility Index (vI)'
slopeTooltip = 'Absolute VMA Slope'
slopePctTooltip = 'VMA Slope (%)'
priceDiffTooltip = 'Price minus VMA'
priceDiffPctTooltip = 'Price-VMA (%)'
corrTooltip = 'Correlation between Price and VMA'
htfVmaTooltip = 'Higher Timeframe VMA (' + htfInput + ')'
var table infoTable = table.new(tablePosition, 2, 10, border_width = tableBorderWidth)
if barstate.islast
table.cell(infoTable, 0, 0, 'Metric', text_color = tableTextColor, bgcolor = tableHeaderBgColor)
table.cell(infoTable, 1, 0, 'Value', text_color = tableTextColor, bgcolor = tableHeaderBgColor)
table.cell(infoTable, 0, 1, 'VMA', text_color = tableTextColor, bgcolor = vmaCellColor, tooltip = vmaTooltip)
table.cell(infoTable, 1, 1, str.tostring(vma, format.mintick), text_color = tableTextColor, bgcolor = vmaCellColor, tooltip = vmaTooltip)
table.cell(infoTable, 0, 2, 'Trend', text_color = tableTextColor, bgcolor = trendCellColor, tooltip = trendTooltip)
table.cell(infoTable, 1, 2, vma > nz(vma[1]) ? 'Bullish' : vma < nz(vma[1]) ? 'Bearish' : 'Neutral', text_color = tableTextColor, bgcolor = trendCellColor, tooltip = trendTooltip)
table.cell(infoTable, 0, 3, 'vI', text_color = tableTextColor, bgcolor = viCellColor, tooltip = 'Volatility Index (vI)')
table.cell(infoTable, 1, 3, str.tostring(vI, format.mintick), text_color = tableTextColor, bgcolor = viCellColor, tooltip = 'Volatility Index (vI)')
table.cell(infoTable, 0, 4, 'Slope', text_color = tableTextColor, bgcolor = slopeCellColor, tooltip = slopeTooltip)
table.cell(infoTable, 1, 4, str.tostring(vmaSlope, format.mintick), text_color = tableTextColor, bgcolor = slopeCellColor, tooltip = slopeTooltip)
table.cell(infoTable, 0, 5, 'Slope %', text_color = tableTextColor, bgcolor = slopePctCellColor, tooltip = slopePctTooltip)
table.cell(infoTable, 1, 5, str.tostring(slopePct, format.mintick) + '%', text_color = tableTextColor, bgcolor = slopePctCellColor, tooltip = slopePctTooltip)
table.cell(infoTable, 0, 6, 'Price-VMA', text_color = tableTextColor, bgcolor = priceDiffCellColor, tooltip = priceDiffTooltip)
table.cell(infoTable, 1, 6, str.tostring(priceDiff, format.mintick), text_color = tableTextColor, bgcolor = priceDiffCellColor, tooltip = priceDiffTooltip)
table.cell(infoTable, 0, 7, 'P-VMA %', text_color = tableTextColor, bgcolor = priceDiffPctCellColor, tooltip = priceDiffPctTooltip)
table.cell(infoTable, 1, 7, str.tostring(priceDiffPct, format.mintick) + '%', text_color = tableTextColor, bgcolor = priceDiffPctCellColor, tooltip = priceDiffPctTooltip)
table.cell(infoTable, 0, 8, 'Corr', text_color = tableTextColor, bgcolor = corrCellColor, tooltip = corrTooltip)
table.cell(infoTable, 1, 8, str.tostring(corr, format.mintick), text_color = tableTextColor, bgcolor = corrCellColor, tooltip = corrTooltip)
table.cell(infoTable, 0, 9, 'HTF VMA', text_color = tableTextColor, bgcolor = htfVmaCellColor, tooltip = htfVmaTooltip)
table.cell(infoTable, 1, 9, str.tostring(htfVma, format.mintick), text_color = tableTextColor, bgcolor = htfVmaCellColor, tooltip = htfVmaTooltip)
vmaRising = vma > nz(vma[1])
bullishSignal = not vmaRising[1] and vmaRising
bearishSignal = vmaRising[1] and not vmaRising
alertcondition(bullishSignal, 'Bullish Trend Change', 'VMA is now bullish')
alertcondition(bearishSignal, 'Bearish Trend Change', 'VMA is now bearish')
extremeSlopeAlert = math.abs(slopePct) >= extremeSlopeThreshold
alertcondition(extremeSlopeAlert, 'Extreme Slope', 'VMA slope is extreme')
alertcondition(corr < lowCorrThreshold, 'Low Correlation Alert', 'Low correlation between Price and VMA')
alertcondition(ta.crossover(vma, htfVma), 'VMA Crosses Above HTF VMA', 'VMA crossed above HTF VMA')
alertcondition(ta.crossunder(vma, htfVma), 'VMA Crosses Below HTF VMA', 'VMA crossed below HTF VMA')
var label vmaLabel = na
if barstate.islast
label.delete(vmaLabel)
vmaLabel := label.new(x = bar_index, y = vma, style = label.style_label_left, text = 'VMA-33: ' + str.tostring(vma, format.mintick), color = trendColor, textcolor = color.white, size = size.normal)
vmaLabel
How to Apply an Indicator Code in Pine Script on TradingView
Follow this step-by-step guide to apply an indicator code in Pine Script on TradingView:
Step 1: Log in to TradingView
Visit TradingView and log in to your account.
If you don’t have an account, create one by clicking on Sign Up.
Step 2: Open the Chart
Click on the “Chart” option in the top navigation bar.
A default chart will load, which you can use to apply your indicator.
Step 3: Open Pine Script Editor
At the bottom of the chart, you’ll see a tab labeled “Pine Script Editor”. Click on it.
If it’s not visible, right-click on the blank area below the chart and enable “Pine Script Editor”.
Step 4: Paste Your Indicator Code
Copy the indicator code you want to use.
Paste the code into the Pine Script Editor.
Step 5: Save the Script
Click the “Save” button at the top of the editor.
Enter a name for your script (e.g., “My Custom Indicator”) and save it.
Step 6: Add the Indicator to the Chart
After saving, click the “Add to Chart” button.
The indicator will now appear on your chart.